We can use RxBinding. This example could help you.
https://github.com/JakeWharton/RxBinding
//Validate username field
Observable<Boolean> usernameObservable = RxTextView.textChanges(usernameEditText)
.map(username -> StringUtils.isNotBlank(username) && Validators.validateUsername(username.toString())).skip(1);
//Validate password field
Observable<CharSequence> passwordObservable = RxTextView.textChanges(passwordEditText).skip(1);
//Validate confirm password field
Observable<CharSequence> confirmPasswordObservable = RxTextView.textChanges(confirmPasswordEditText)
.skip(1);
//Validate password matches field
Observable<Boolean> passwordMatcherObservable = Observable.combineLatest(passwordObservable, confirmPasswordObservable,
(password, confirmPassword) -> password.toString().equals(confirmPassword.toString())).skip(1);
Observable.combineLatest(passwordMatcherObservable, usernameObservable,
(passwordMatch, isUsernameValid) -> passwordMatch && isUsernameValid)
.distinctUntilChanged()
.subscribe(valid -> createAccountBtn.setEnabled(valid));
https://medium.com/@goofyahead/debouncing-edittext-with-rxandroid-894e15da1012
Debouncing EditText with RxAndroid
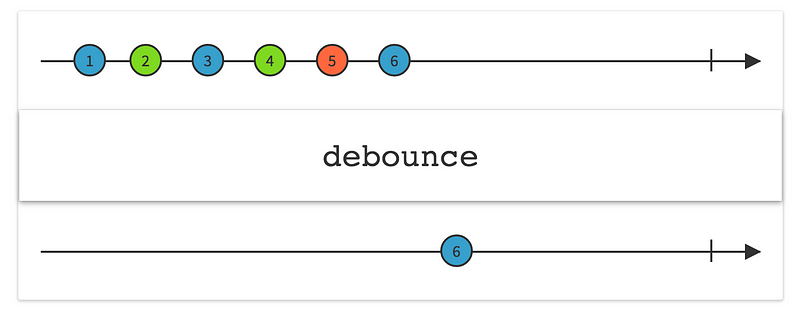
Typical case, several clics on a button, typing fast on a search box… Those are problems that we have to take care on front end for a better smooth UX.
So lets implement it on android, its also a perfect time to start with Rx and observable patterns.
First dependencies needed:
project gradle
...
dependencies { classpath 'com.android.tools.build:gradle:2.2.0-rc1' classpath 'me.tatarka:gradle-retrolambda:3.2.5'
...
App gradle
//on top of app graddle apply plugin: 'me.tatarka.retrolambda'
...
compile 'io.reactivex:rxandroid:1.2.1' compile 'io.reactivex:rxjava:1.1.6' compile 'com.jakewharton.rxbinding:rxbinding:0.4.0' compile 'com.jakewharton.rxbinding:rxbinding-design:0.4.0'
// these are for retrolambda and streams api compile 'com.annimon:stream:1.1.2'
These are retrolambda and stream api for android, Rx java dependencies, Rx for android and UI bindings from jakewharton 🙂
Thats nice but show me the code!
Observable<String> obs = RxTextView.textChanges(searchBox).filter(charSequence -> charSequence.length() > 3).debounce(300, TimeUnit.MILLISECONDS).map(charSequence -> charSequence.toString()); obs.subscribe(string -> { Log.d(TAG, "debounced " + string); startIntentService(prefs.getLastLocation().getCountry() + ", " + string); });
Yay! with just a few lines we got our debounced EditText that will save us some calls to our service and network calls 🙂